Photo by Fabian Grohs on Unsplash |
So, there has been a lot of buzz surrounding typescript for quite a while now. Especially since Angular started using TypeScript as its primary language. Now, you might think: Angular was developed by Google, a company that has pretty smart programmers (top 4%, some might say). If they choose TS over JS, there must be a good reason!
Well, you are right! and here are a few reasons to learn TS, ASAP!
- TypeScript is the future of JavaScript (some might disagree!)
- Typescript code is like a well-documented code, hence, it’s easier to understand.
- It has classes, interfaces, generics, etc. like any other object-oriented programming language.
This might look obvious, but if it was JavaScript, there is almost no easy way to know “what kind of variables you should use when calling this function?” or “what will it return, a number or a string?” And thus, leading to potential bugs in the end.
Now, what if after reading the function above, you still make a mistake and try passing a string as an argument to the above function.Well, in the case of JS, you will only know it when it's already too late.However, our friendly TS will let us know right away.If you don’t believe me, see below:
Types
As the name suggests, TypeScript has types! We have already seen the type number and string above. What else…
Classes
Have a look at the code below, don’t you think this kind of code is easier to comprehend and less error-prone.
Code Explanation: Think of the “Employee” class as a blueprint. This piece of code, although pretty basic, explains some of the very important concepts of typescript. Also, note that the salary variable is private. That means we cannot directly access this variable. See the snapshot below:
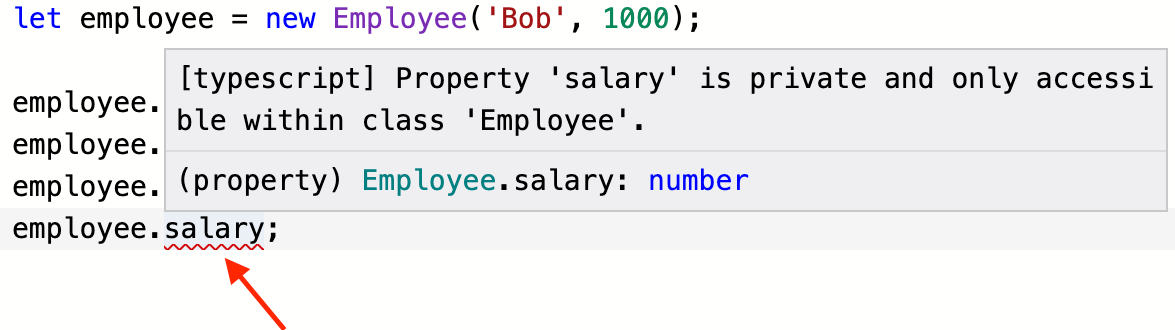
For more information on classes (typescriptlang.org): classes.
Interfaces: create your own custom-types
We already know that we can use types like string, number, boolean, etc. What if we want to create our own custom types for enforcing checks in our code. Luckily we can use interfaces for that!
Interfaces can be really useful and are heavily used in frameworks like Angular/React. We can also use “model” classes or “types” depending on the use-case.
For more information on interfaces (typescriptlang.org): interfaces.
Generics
Generics is a very simple, yet powerful feature of typescript. Using generics is widely appreciated while working on services/APIs that other developers might use, especially, open-source ones. It helps to know what kind of data to expect and hence, resulting in reduced errors and confusion.
Resources
You can find the code in this article here: Link to the Github gists.
Other resources: Official typescript handbook.
Thanks for reading. If you have any questions, feel free to leave a response.
Comments
Post a Comment